So, you're new to coding and wondering where to begin, right? The world of programming can feel like venturing into a dense forest without a map. But hey, it doesn't have to be that way! Taking it one step at a time can make everything much clearer.
First off, you've got to understand the problem you're trying to solve. Picture this like assembling IKEA furniture—reading the instructions before diving in helps a ton, trust me. So, spend some time figuring out what the problem actually is. It's all about asking the right questions.
Once you know what's what, it's time to plan your solution. Imagine you're about to cook a meal. You wouldn't just start tossing ingredients into a pot without following a recipe, right? Coding works similarly. Outline your steps before you start typing away. You'll thank yourself later when things get tricky.
- Understanding the Problem
- Planning the Solution
- Writing the Code
- Testing the Code
- Debugging
- Optimizing the Code
- Reviewing and Learning
Understanding the Problem
Diving into coding isn't just about writing lines of code. Before fingers hit the keyboard, you need to get a solid grasp on the problem at hand. Think of it like solving a puzzle; you’ve got to see the big picture first.
Start by dissecting the problem. Ask yourself questions like: What exactly is being asked? What are the constraints? Picture you're designing a simple calculator. You need to know whether it should just add and subtract or handle complex operations like factorials. Getting these details straight saves you from unnecessary headaches later.
Another pro tip is to break down the problem into smaller chunks. Tackling a massive task all at once can feel overwhelming, so break it into manageable pieces. If you're building an app, maybe start with the login screen before moving onto other features.
Rather than diving headfirst, research similar problems. Online forums like Stack Overflow or GitHub can be gold mines for beginners. See how others approached similar issues, learn from their successes, and avoid their mistakes.
Don’t forget about documenting your understanding. Write it down or sketch it out. This helps clarify your thoughts and gives you a reference point. When you've got a clear understanding, take a deep breath and get ready to move forward with planning your solution.
Planning the Solution
Alright, so you've got a good grip on the problem you're tackling. What's next? Time to plan your solution. Think of this as drawing the blueprint before building a house. Without a solid plan, you might find yourself in a sea of code with no life raft in sight.
Begin by breaking down the problem into smaller, more manageable pieces. Imagine a coding project as a pie. You wouldn't try to eat the whole pie at once, right? Slice the pie into pieces—you'll handle these one by one.
Flowcharts and Pseudocode
Mapping out your plan with flowcharts or writing pseudocode can be incredibly helpful. Flowcharts give you a visual overview of the sequence your code needs to follow. Pseudocode, on the other hand, looks like code but using plain language, giving you a step-by-step guide.
Choosing the Right Tools
Once you've got your plan laid out, consider the tools and languages you'll need. Different programming languages offer unique strengths. If you're learning to code, you might start with Python because of its simplicity and readability. Got performance-heavy tasks? Perhaps C++ is your thing.
Setting Goals
Establish clear goals and timelines for each part of your project. This keeps you on track and helps manage progress. Think of it like a road trip—having a map and timeline ensures you reach your destination without unnecessary detours.
Example: Building a Simple Calculator
Let's say you want to build a simple calculator. Broken down, you might focus on basic operations like addition, subtraction, multiplication, and division. The plan could look something like this:
- Accept user input.
- Determine the operation type (add, subtract, multiply, or divide).
- Perform the corresponding calculation based on inputs.
- Display the result to the user.
Stick to the plan and you'll have a solid foundation to guide your coding steps forward. Remember, investing time in planning saves you debugging headaches later.
Writing the Code
Alright, you've done your planning, and now it's time to jump into the actual coding steps. Think of this phase as getting your hands dirty in the best way possible. It's where your ideas come alive on screen. But don't just start typing; there's a method to the madness!
Choose Your Language
Before hitting those keys, decide on the programming language that'll best suit your project. Languages like Python are super beginner-friendly, while JavaScript is great for web development. Choosing the right tool makes coding less of a headache.
Start Simple
Begin with the simplest code that solves a basic part of your problem. Let's say you're coding a calculator. Start by writing the part that adds two numbers. Don't feel pressured to get everything right at once.
- Open your text editor — even a basic one like Notepad can work.
- Set up your environment if needed. Install any necessary software or libraries.
- Write the simplest version, like a "Hello World" program, to ensure everything's working correctly.
Keep Code Organized
Remember, you're creating something you'll have to revisit. Use comments to remind yourself (and others) what different parts of the code do. For instance:
# This function adds two numbers
def add_numbers(a, b):
return a + b
Test as You Go
Don't wait until the end to check if your code works. Test each piece as you write it. This way, if something goes wrong, you know exactly where to look. It's like checking your reflection during a painting class to avoid massive mistakes.
By the way, did you know that according to a 2023 survey, developers spend almost 50% of their time debugging? Testing as you go can save you a lot of time and prevent headache-inducing bugs.
Iterate and Improve
Once you've got the basic code running, it's time to loop back and polish your work. Refactor your code, which means cleaning it up, making it more efficient, and ensuring it's easy to understand. Good coding is like good writing—concise and clear.
And there you go! With these coding steps, you're well on your way to writing solid code. Remember, patience and practice are your best pals in this journey.
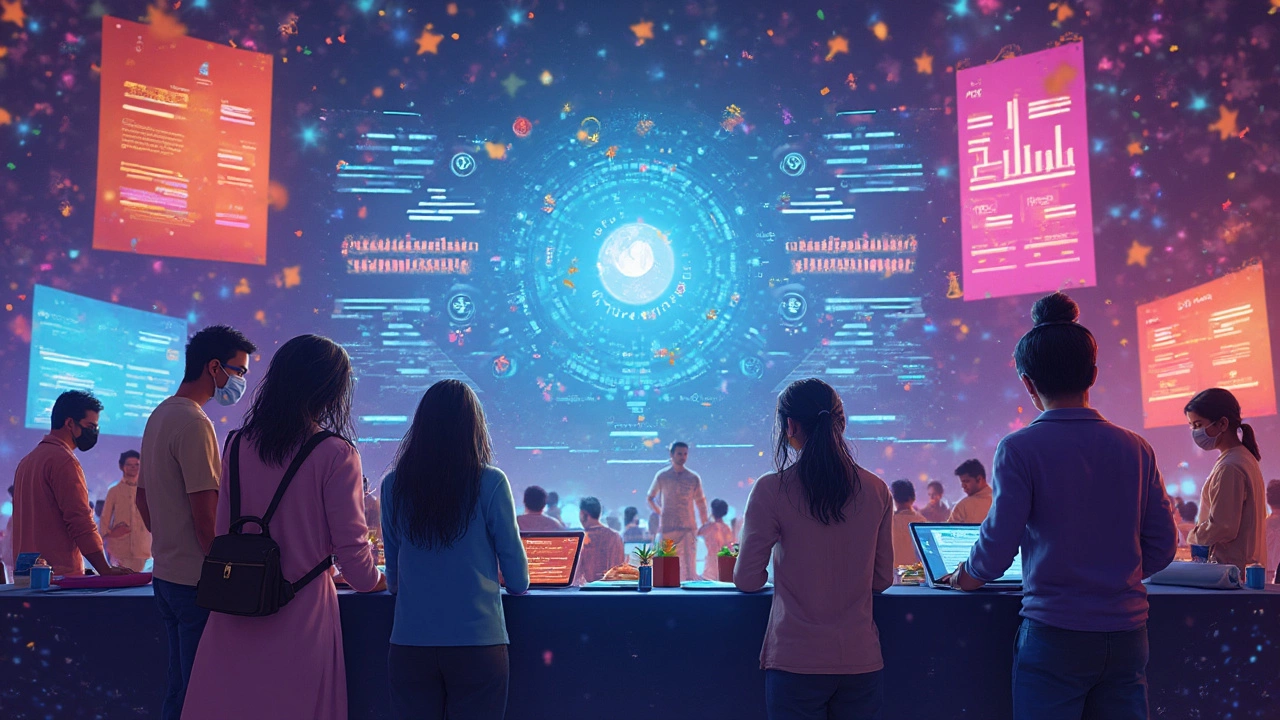
Testing the Code
Alright, you've written your code, and now comes the crucial part—making sure it actually works. Think of this like giving your new bike a test ride before heading off on a long trip. You need to know everything's running smoothly.
To start, run your code and see if it behaves as expected. This is often referred to as unit testing, where you check small parts of your code individually. If you’re coding a series of tasks, make sure each task is completed correctly before moving on. It’s like checking the wheels, brakes, and handlebars individually on that bike.
Common Testing Techniques
- Manual Testing: This is simply running your code and manually checking the output. It's like doing a quick scan of the code by hand to make sure everything’s there.
- Automated Testing: Use scripts or testing frameworks to run your code. This is handy for large projects or ongoing development. Frameworks like JUnit for Java or PyTest for Python can save you loads of time.
When you’re testing the code, pay careful attention to edge cases. These are instances where inputs are unusual or extreme, and they're often where bugs love to hide. For example, what happens if someone tries to log into an app with an empty password? Testing should reveal issues like these before your users do.
Testing Method | Best For | Example Tools |
---|---|---|
Manual Testing | Small Projects | None needed |
Automated Testing | Large Projects Frequent Changes | JUnit, PyTest, TestNG |
Debugging often begins here, too. If something doesn’t work, backtrack and see where things went awry. Errors can often teach you more about coding than successes. Remember, bugs are inevitable, but testing gives you a chance to squash them before they become real problems!
Debugging
Alright, you've written your code and given it a spin only to find things aren't quite working—bummer! But don’t worry, debugging is a crucial part of the coding steps and happens to everyone. In fact, some say developers spend more time debugging than actually writing code.
Let's break down debugging into a few manageable actions. First, you’ve got to identify what’s going wrong. Be your own detective! Start by running your code and carefully watching for any errors or unexpected results. Pro tip: Read those error messages; they're like breadcrumbs telling you where it all went south.
Strategies to Debug
Once you've spotted the issue, let's talk strategies. One of the go-to methods is the "Rubber Duck Debugging" technique. Yes, it sounds silly, but explaining your code to a rubber duck (or a pal if you prefer) can make the solution pop into your mind. The act of talking it out often reveals hidden problems.
Also, consider using a debugger tool—most Integrated Development Environments (IDEs) offer this feature. Debuggers let you walk through your code step-by-step, check variable states, and watch the flow of execution.
- Print Statements: When in doubt, print it out! Adding print statements in key parts of your code can reveal what the program is actually doing versus what you think it should do.
- Breakpoints: Utilize breakpoints to pause code execution. This helps inspect the program state at specific moments in the process.
Common Errors
Some common culprits among newbie coders include off-by-one errors, typos, and mixing up data types. Watch out for these pitfalls!
Finally, remember that debugging can feel frustrating, but it's where you polish your skills. As you go through the process, you're not just fixing issues—you're learning to prevent them in the future. And hey, every bug squashed is another step towards coding greatness!
Common Debugging Tools | Platforms |
---|---|
Chrome DevTools | Web Development |
GDB | C/C++ |
ADB | Android |
Optimizing the Code
After you've got your code up and running, it's time to make it shine by optimizing. Now, what does that mean? Well, optimizing your code is like tuning an engine for better performance. You're making sure it runs fast, uses fewer resources, and can handle more tasks without breaking a sweat.
Why Bother with Optimization?
Think about it: no one wants a slow program. It's like watching paint dry. Everyone loves a snappy, efficient application. If you're serious about programming basics, you need to prioritize optimization. This minimizes load times, saves on server costs, and generally provides a better experience for users.
How to Optimize?
- Clean Up Your Code: Strip out redundant lines and comments that are no longer needed. Like spring cleaning, you'll make everything neater.
- Use Efficient Algorithms: Choose algorithms that do the job with the least effort, like sorting algorithms for data organization. The right choice can drastically reduce processing time.
- Mind Your Memory: Be mindful of memory usage. Free up memory when it’s no longer needed and avoid unnecessary data duplication.
Practical Example
Let's say you're coding a sorting function. Instead of your basic bubble sort—which works but isn’t super-efficient—try a quick sort. It divides and conquers, getting things sorted in no time.
Do You Need to Optimize All the Time?
Not necessarily. Sometimes, getting the code to work is enough, especially if it's a personal project or a quick prototype. But in a production environment where performance matters, optimization is key.
Remember, knowing when and how to optimize is a crucial part of your coding guide. It's like knowing when to sprint and when to jog in a marathon.
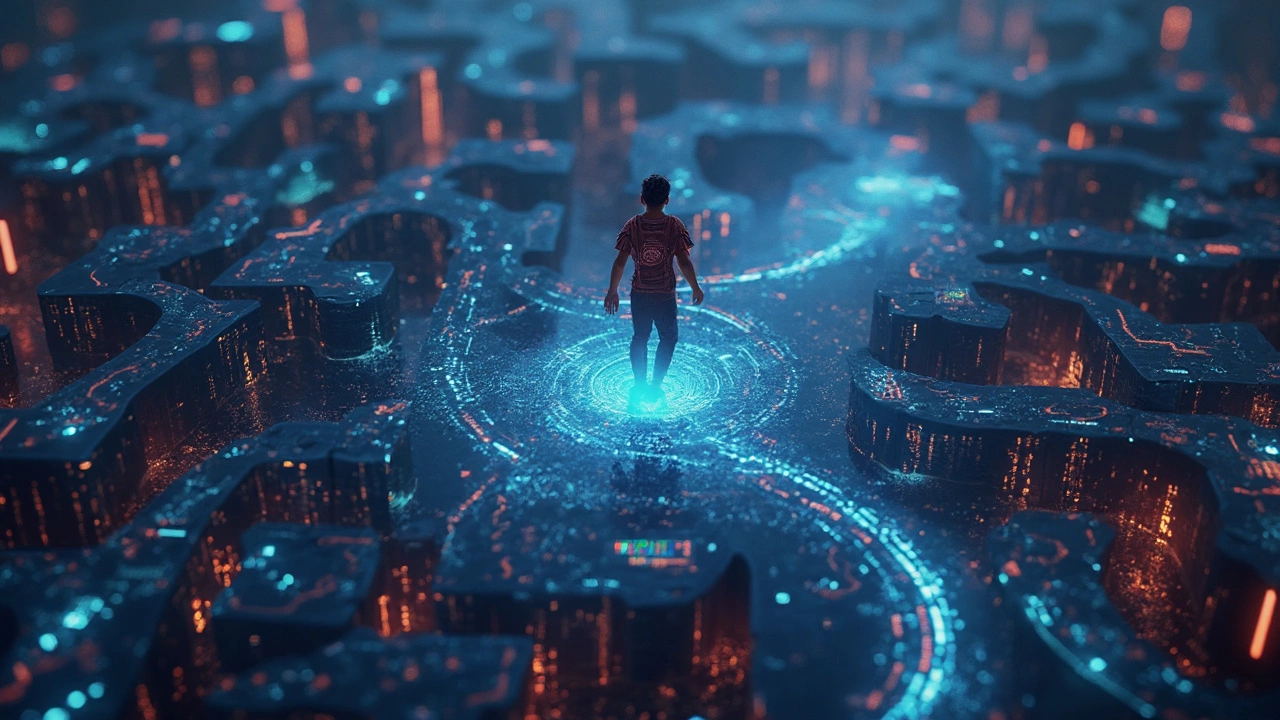
Reviewing and Learning
Alright, you've built and tested your project. But what now? The journey doesn't end after writing the last line of code - it is crucial to step back and review. This is where the magic happens.
First, give your code a good once-over. Look for areas to improve. Perhaps there's a more efficient way to get the same result—after all, clean and optimized coding should be a goal. Look for repeated patterns or unnecessary complexity and simplify where you can.
Why Reviewing Matters
Reviewing is critical because it often reveals overlooked mistakes. As the famed computer scientist Donald Knuth once said,
"Beware of bugs in the above code; I have only proved it correct, not tried it."
This means, no matter how confident you are in your solution, there's always room for scrutiny. Testing will catch obvious errors, but reviewing helps in maintaining clarity and readability.
Learn from Each Mistake
Mistakes are going to happen. But that's not a bad thing. Instead, consider each bug or failed attempt as a learning opportunity. Jot down what went wrong and what you did to fix it. This habit can solidify your understanding and prevent the same errors in the future.
Keep Abreast with Trends
The world of coding is dynamic, and staying updated with new trends and technologies is essential. Join coding communities, follow influential programmers on social media, or even read up on the latest programming books. Make sure you're regularly reflecting on your work strategies and adapting as needed.
Here’s a quick table highlighting some programming languages popularity to consider:
Language | Popularity % |
---|---|
Python | 31% |
JavaScript | 25% |
Java | 15% |
Learning is a continuous process. By reviewing and learning, you make yourself better, whether it's enhancing basic programming techniques or diving deeper into the nitty-gritty of coding. So, always make time to revisit what you've done and explore new skills.
Write a comment